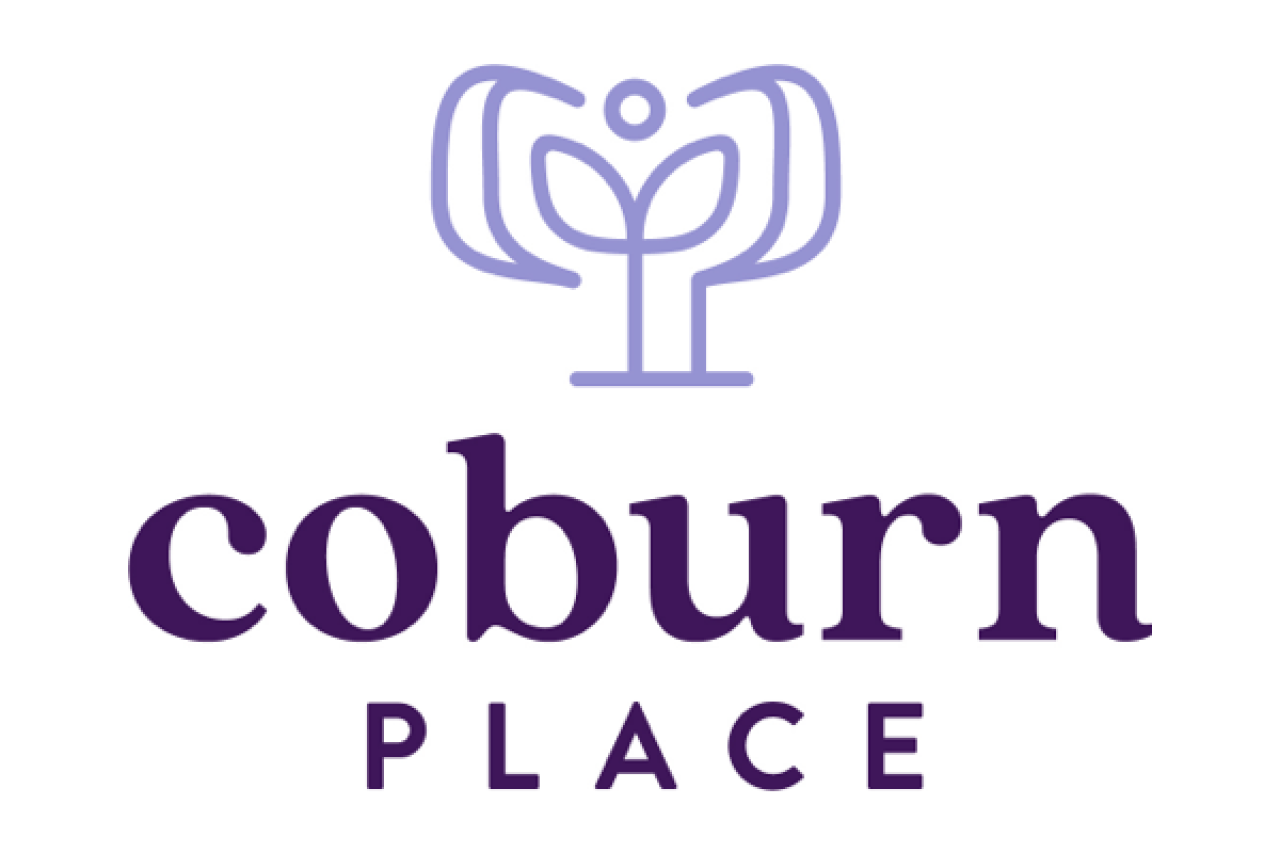
In FileMaker Web Apps Part I of this series we focused on how you can use Vue.js to extend FileMaker using web viewers. In this article we'll focus on how you can build robust web portals that use FileMaker as the backend and Vue.js as the frontend. This will allow you to build web contact forms, customer portals, e-commerce sites, etc, using data in your FileMaker system.
Web portals using FileMaker's Data API are a great fit when you have hundreds or thousands of users or if the users are anonymous. In this article we'll go over how to build a very simple customer portal that allows users to log in and see their invoices. We'll build our web app using Nuxt.js which is just an SEO-friendly version of Vue. All of the source code will be available in the FileMaker sample file, so make sure to download it to get access to all the code.

Getting Started
To get started install Node.js and NPM if you don't already have them on the web server, at minimum NPM 5.2.0. Next, open either command prompt in Windows or terminal on a Mac and type the following command.
$ npx create-nuxt-app <project-name>
Fill in the project name, description, author name, and NPM as the package manager. Then you can pick your favorite UI framework. In this example, we will be using Buefy to get up and going quickly with a huge library of easy-to-use components.
The CLI will then ask you what backend if any you want to use. In our example, we'll be using Express. Then pick your testing framework of choice and the rendering mode you want, either SSR (Server Rendered) or SPA (Single Page Application). In our example we'll be using SSR with Nuxt. Nuxt makes server-side-rendered Vue apps easier to build, and rendering on server is important for SEO because many web crawlers rank a site poorly that don't work properly without JavaScript. While Google's Googlebot can render JavaScript, it does so at a much slower pace than a traditional static website.
Finally, include Axios which is what we will use to make HTTP calls to the Data API.
Backend
The first thing we'll work on is our backend. It's important to know that you can use whatever backend you want, any will work with the FileMaker Data API. Quite often we will use PHP for our backend, but PHP is not well suited for server-side rendering JavaScript, so we will be using Express today. For our example our backend needs the ability to do a few things:
Authenticate and log in a user
Log out and destroy a user's session
List the logged-in users invoices
Load an invoice with all the invoice line items
In order to make any of these calls, we'll need the ability to generate an auth token. To do so, first update the nuxt.config.js file with info about the host, file, username, and password. Because Nuxt.js is isomorphic (aka universal), we'll have access to settings stored here on the server and client.
//nuxt.config.js
module.exports = {
...
fmDatabase: "<filename>",
fmHost: "https://<<host>>.com",
fmUsername: "<username>",
fmPassword: "<password>",
...
}
We'll need the ability to store session data on the server so we can remember who is logged in and keep track of people's auth tokens. To do this install the express-session module from the command line.
$ npm install express-session
Then include the module in your server/index.js file and configure your Express app to use the session module. Make sure to set the secret to something secure.
const session = require('express-session')
app.use(session({
secret: 'super-secret-key',
resave: false,
saveUninitialized: false,
cookie: {}
}))
After you've done that you'll have access to the session object inside your route handlers.
Routing
Nuxt handles routing a bit differently than a typical Vue + Vue Router setup. Instead of manually creating your routes, you simply add your files to the pages directory and each one will get added to the router automatically. If you follow Nuxt's default naming configuration, Nuxt will automatically set up basic routes for you, and the routes will work with or without JavaScript. Instead of using <a></a> tags in your system, you'll want to use the <nuxt-link></nuxt-link> component that will ensure your pages change without a full reload.
Another change from Vue Router is that you'll use middleware to route guards to prevent people from accessing data they shouldn't have access to. In our case we'll add a protected.js file to our middleware folder that will ensure our user is logged in before we show them an invoice.
To set up our pages to call the new protected middleware we'll add a middleware property to our invoice pages Vue instance with an array of all the middlewares the page should load.
//pages/invoices/index.vue
export default {
name: "invoices",
middleware:['protected'],
...
}
Application State
Nuxt uses Vuex behind the scenes to handle global application state which makes it easy to keep things in-sync. The one problem we have right now though is that each page reload clears out the Vuex store. Luckily we're already saving our needed data in session variables with our Express backend.
Nuxt also provides a way to load data into the store on first page load, via the nuxtServerInit action in our store.js file. The nuxtServerInit function has access to both our express request object and to our store, so we can take data from our session and update our user state using a SET_USER mutation. Any other application state could also be saved to the express session if needed.
Conclusion
Building a web portal that pulls data from FileMaker might seem like a daunting task, but the reward is well worth it. It allows you to extend your system to reach a much larger audience than with FileMaker alone. FileMaker, Data API and Vue.js are perfect for web portals, contact forms, registration sites, and even pulling real-time inventory data. Contact us today if you would like help building your own FileMaker web portal.
Did you know we are an authorized reseller for Claris FileMaker Licensing?
Contact us to discuss upgrading your Claris FileMaker software.
Download the FileMaker Web Portals With Vue.Js File
Please complete the form below to download your FREE FileMaker file.